Java: Optimization of 1000 if-else statements

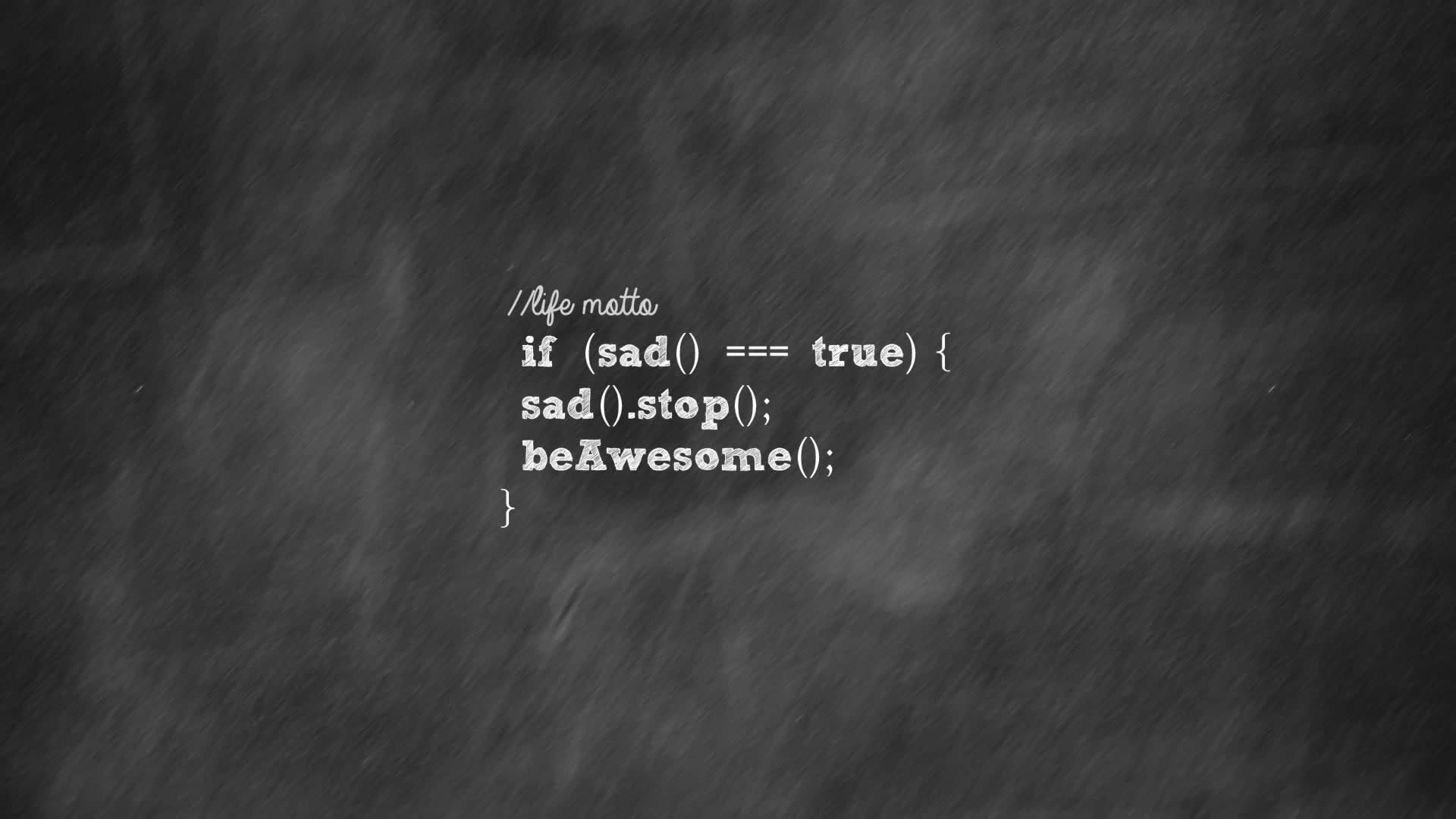

The primary challenge is writing 1000 lines of if-else statements. At a rate of 100 lines per day, this would take approximately 10 days to complete. Additionally, processing each request would involve executing hundreds of conditional checks. This approach would not only complicate code maintenance but also significantly degrade system performance. It is evident that the interviewer is seeking more than standard textbook responses. This question serves as a scenario-based evaluation of a programmer’s ability to optimize complex logic and demonstrate technical improvisation skills. While my colleague suggested the strategy pattern, it is neither the perfect nor the sole solution. Other design patterns, such as the chain of responsibility pattern, also present challenges. Thus, it is crucial to analyze the specific problem to determine the most suitable approach.
Strategy Pattern
While the strategy pattern can enhance code elegance, it also presents several challenges: 1. Class Bloat: For a large number of if-else branches, such as 1000, this would result in 1000 strategy classes, leading to excessive class proliferation, which becomes increasingly complex and difficult to manage over time. 2. Nested If-Else Statements: In cases of multiple layers of nested if-else statements, the strategy pattern may not be effective. The primary advantage of the strategy pattern is that it decouples code efficiently and is suitable for scenarios involving diverse logics and algorithms. However, it is not appropriate for situations involving a large number of if-else statements.
Variant of the Strategy Pattern
package hr.from.juricamigac.core;
import java.util.HashMap; import java.util.Map; import java.util.Objects; import java.util.Optional; public class Main { public static void main(String[] args) { final Map<String, Runnable> actionMap = new HashMap<>(); actionMap.put("firstCondition", () -> { System.out.println("Execution of the First condition"); }); actionMap.put("secondCondition", () -> { System.out.println("Execution of the Second condition"); }); actionMap.put("thirdCondition", () -> { System.out.println("Execution of the Second condition"); }); final String condition = "secondCondition"; Optional.of(condition) .map(actionMap::get) .filter(Objects::nonNull) .ifPresent(Runnable::run); } }
This approach separates the business logic code, simplifying individual class code and preventing the proliferation of strategy implementation classes. However, with numerous condition mappings, a single class can still become bloated and difficult to maintain. In this example, thread asynchronous execution is employed. The execution logic code can also be stored in other classes or databases, then loaded and executed using reflection or dynamic compilation.
Multi-level Nesting Optimization
The above two solutions might not work for nested structures. For such layered judgments, optimization is possible:
if(object != null) { if(object.getCarModel() != null) { if(object.getCarModel().getCarPackage() != null) { if(object.getCarModel().getCarPackage().getPackagePriceModel() != null) { ... business logic ... } } } }
Too many if-else statements? See how you can easily eliminated them with Java 8!
Optional.ofNullable(object) .map(Object::getCarModel) .filter(Objects::nonNull) .map(CarModel::getCarPackage) .filter(Objects::nonNull) .map(CarPackage::getPackagePriceModel) .filter(Objects::nonNull) .ifPresent(packagePriceModel -> { ... business logic ... });
Using Ternary Operators
If the conditions are not numerous, and there are only 2 or 3, you can use ternary operators to simplify if-else branches.
For example, the following code:
String brand; if (condition1) { brand= "brand1"; } else if (condition2) { brand= "brand2"; } else { brand= "brand3"; }
Can be simplified to one line using a ternary operator:
final String brand= brand1 ? "brand1" : (brand2 ? "brand2" : "brand3");
It is not recommended to use this for more than 3 conditions, as it would significantly reduce code readability.
Using Enums
Enum types can represent a fixed set of values, such as days of the week, months, or colors. They offer a more concise and readable way to handle related constants, ensuring type safety and reducing errors associated with invalid values. By using enum types, code becomes more maintainable and easier to understand. They help avoid the pitfalls of using primitive types or strings for constant values, which can lead to inconsistencies and bugs. Additionally, enum types can include fields, methods, and constructors, allowing for more complex data structures and behaviors to be encapsulated within the enum, further enhancing code organization and clarity.
public class Main { public static void main(String[] args) { final EnginePart cylinder = EnginePart.CYLINDER; final Optional<EnginePart> piston = EnginePart.getEnginePartByName("Piston"); final String firstMsg = String.format("%s is an engine!", cylinder.getEnginePartName()); System.out.println(firstMsg); piston.ifPresent(enginePart -> System.out.println(enginePart + " is also in the engine!")); } }
enum EnginePart { CYLINDER("Cylinder"), CYLINDER_HEAD("Cylinder Head"), PISTON("Piston"), PISTON_RING("Piston Ring"), CRANKSHAFT("Crank Shaft"); private final String name; EnginePart(final String enginePart) { this.name = enginePart; } public String getEnginePartName() { return this.name; } public static Optional<EnginePart> getEnginePartByName(final String partName) { return Optional.of(EnginePart.values()) .map(Arrays::asList) .stream() .flatMap(List::stream) .filter(enginePart -> StringUtils.equalsIgnoreCase(enginePart.getEnginePartName(), partName)) .findFirst(); } }
Early Return
To optimize business logic, begin by analyzing and sorting the if-else statements based on their execution frequency, arranging them in descending order. This prioritization allows you to evaluate the most frequently occurring conditions first. By addressing the most common cases early, you can use return statements to exit the sequence before evaluating less likely conditions. This approach minimizes the number of checks performed for each request, enhancing overall system performance. By focusing on the most frequent scenarios, you can streamline the decision-making process, reducing the computational overhead associated with evaluating every possible condition. This method also improves the readability and maintainability of the code, as it highlights the primary use cases upfront, making the logic easier to follow. Moreover, this strategy can significantly decrease the execution time, particularly in systems with a large volume of requests or complex business rules. By avoiding unnecessary evaluations, you ensure that the system responds more quickly and efficiently. In conclusion, sorting if-else statements by their execution frequency and using return statements to bypass unnecessary conditions is a practical technique for optimizing code performance and maintainability. This targeted approach allows you to handle the most common cases efficiently, ensuring a more responsive and manageable codebase.
if (condition1) { return } if (condition2) { return }
This simple modification can significantly improve system performance. However, it still has the following issues:
Some conditions cannot be sorted by execution frequency due to sequential or mutually exclusive relationships.
When adding a new condition, it might be challenging to immediately determine its execution frequency. Placing it at the end might still affect performance.
This approach does not help with class bloat or code maintenance.
Eliminate Unnecessary If-Else Statements
if (condition) { ... } else { return; }
It can be optimized as follows:
if (!condition) { return; }
Or even:
return !condition
Merge Conditions
When dealing with a large number of if-else statements, such as 1000, it is essential to evaluate whether all of them are truly necessary. Start by examining the conditions to determine if they can be merged or categorized. For instance, similar logic conditions can often be grouped into broader categories, thereby significantly reducing the number of if-else statements. By identifying common patterns or themes among the conditions, you can consolidate them into a single statement or a smaller set of statements. This not only simplifies the code but also makes it more readable and easier to maintain. Grouping conditions can be done by creating higher-level abstractions that encapsulate similar logic. For example, instead of having separate conditions for each day of the week, you could group them under weekdays and weekends if the logic is the same for each group. Similarly, if multiple conditions share the same outcome, they can be combined into a single if-else statement with a logical OR operator. In addition, consider using data structures such as arrays, dictionaries, or lookup tables to handle repetitive conditions more efficiently. This approach can further streamline the code and improve performance by reducing the number of explicit if-else statements. In conclusion, critically assessing the necessity of each if-else statement and exploring opportunities to merge or categorize them can lead to a more efficient and maintainable codebase.
double calculateSalesTax() { if (firstTax > 10) { return 0.24; } if (secondTax > 3) { return 0.24; } if (thirdTaxIsApplicable) { return 0.24; } }
Can be optimized as follows:
double calculateShipping() { if (orderAmount > 1000 || customerLoyaltyLevel > 5 || promotionIsActive) { return 0.5; } }
Summary
Mastering methods to optimize if-else statements is crucial, especially as interviewers often explore this topic in various ways. To effectively address such questions, it’s important to understand the context. Clarify whether the 1000 if-else statements are within a single code block or spread throughout a project, and tailor your response accordingly. Misunderstanding the business scenario can lead to incorrect answers and potential pitfalls during the interview. There are additional techniques applicable to various scenarios, such as using polymorphism, the chain of responsibility pattern, the template method pattern, and others to eliminate or reduce the reliance on if-else constructs. In conclusion, there is no universal method to eliminate if-else statements entirely, nor can they be completely optimized away. In actual development, different methods should be employed based on the specific scenario, often combining multiple approaches for the best results. This holistic approach ensures that the code is efficient, maintainable, and adaptable to changing requirements.